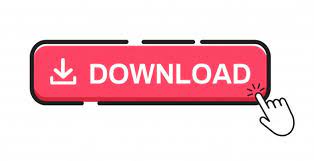
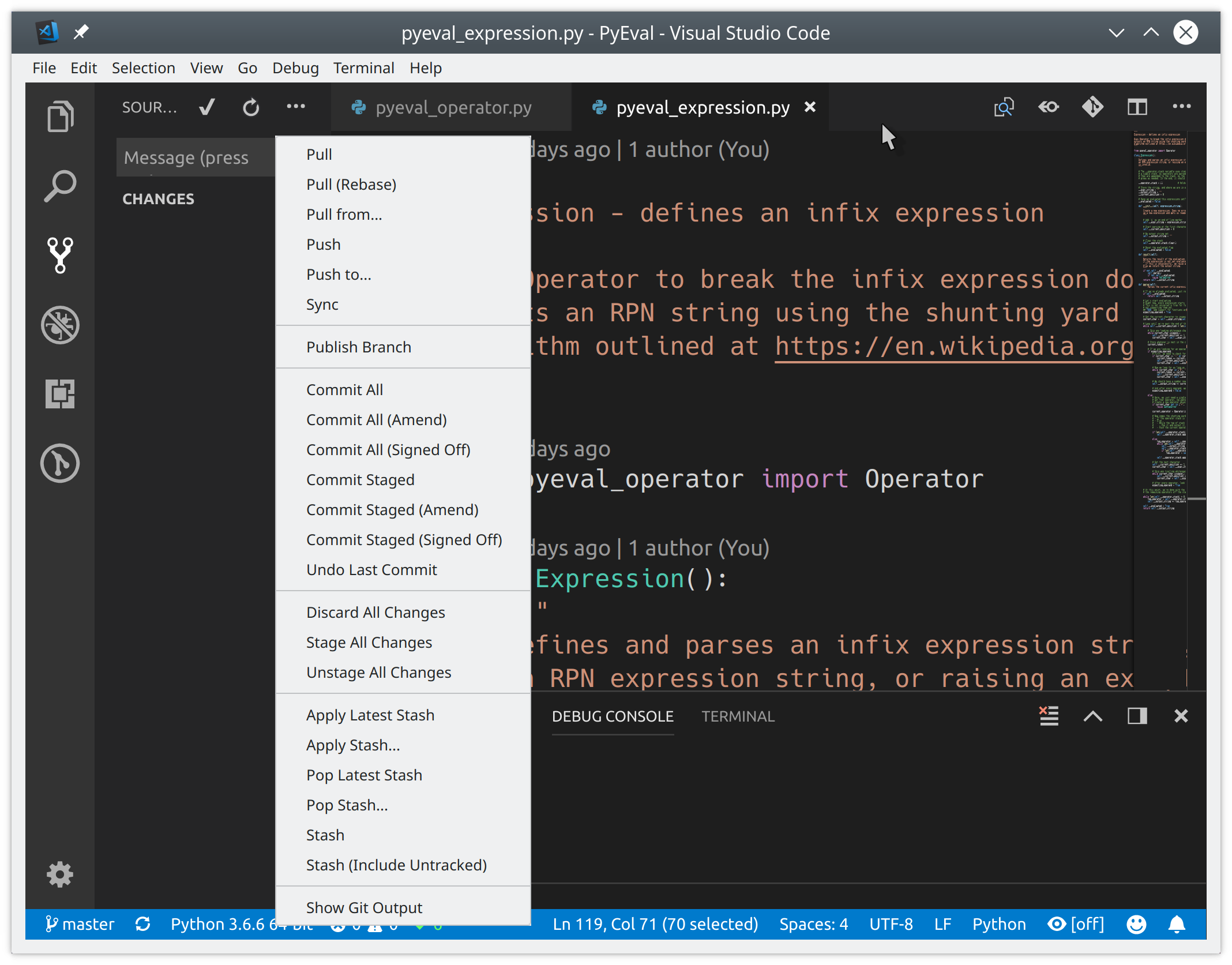
- #Visual studio python tutorial pdf how to#
- #Visual studio python tutorial pdf code#
- #Visual studio python tutorial pdf plus#
Once you've implemented all of the methods correctly, you can try out your implementation by running python runlife.py For your convenience, we have provided two methods in util.py: "createEmptyGrid(rows, cols)" which returns a new grid with the given dimensions in which all cells are set to be dead, and "getNumLiveNeighbors(grid, row, col)" which returns the number of living neighbors in the given cell of the given grid. This is the ultimate test of your understanding of lists and dictionaries, as you'll need to leverage those structures along with the methods you've written to implement the rules of Conway's Game of Life. Sets the cell in the second row and third column to be alive.įinally, implement the "runTimeStep(self)" method. In our case, each cell in our board is a dictionary which maps the string 'alive' into True or False, depending on whether the cell at that position is alive or dead. Instead of being indexed by numbers, dictionaries can be indexed by any type of data, as in: dictionary = 'pi' A dictionary is initialized as dictionary = If a list in Python is similar to an array in Java, then a dictionary in Python is similar to a Map (i.e., HashMap or TreeMap). The element of the list in row r and column c can then be accessed: list In Python, an n-by-m list of zeros (which is similar to an n-by-m array in Java or C) is initialized as list = for y in range(m)]
#Visual studio python tutorial pdf how to#
To do this, you'll need to know how to use Python's list and dictionary data structures, as the board passed into your constructor is a list of dictionaries. Next, implement the methods "isAlive(self, row, col)" which returns True if the cell at the given row and column is alive and False otherwise, "setAlive(self, row, col, tf)" which sets the cell at the given row and column to have the value tf, "getNumRows(self)" which returns the number of rows in the game board, and "getNumCols(self)" which returns the number of columns in the game board. Define instance variables "rows", "cols", and "grid".
#Visual studio python tutorial pdf code#
Now that we know this, write the code for Life's constructor. Thus, instead of needing to define our instance variables outside of the constructor and setting their values inside of it, we can simply write "self.variable = value" in our constructor and have "self.variable" serve as an instance variable which can be used by any other methods in the class. One of the first things you should understand about Python is that, unlike Java, it allows you to both define and set the value of instance variables in the same line. In Python, classes have the following format: class : What you're looking at is a class called "Life". Open the file "life.py" in the "part1" subfolder of the "gameoflife" folder you downloaded. And to just get super meta for a second, a consequence of this is that Game of Life can simulate ITSELF! (#Conwayception)
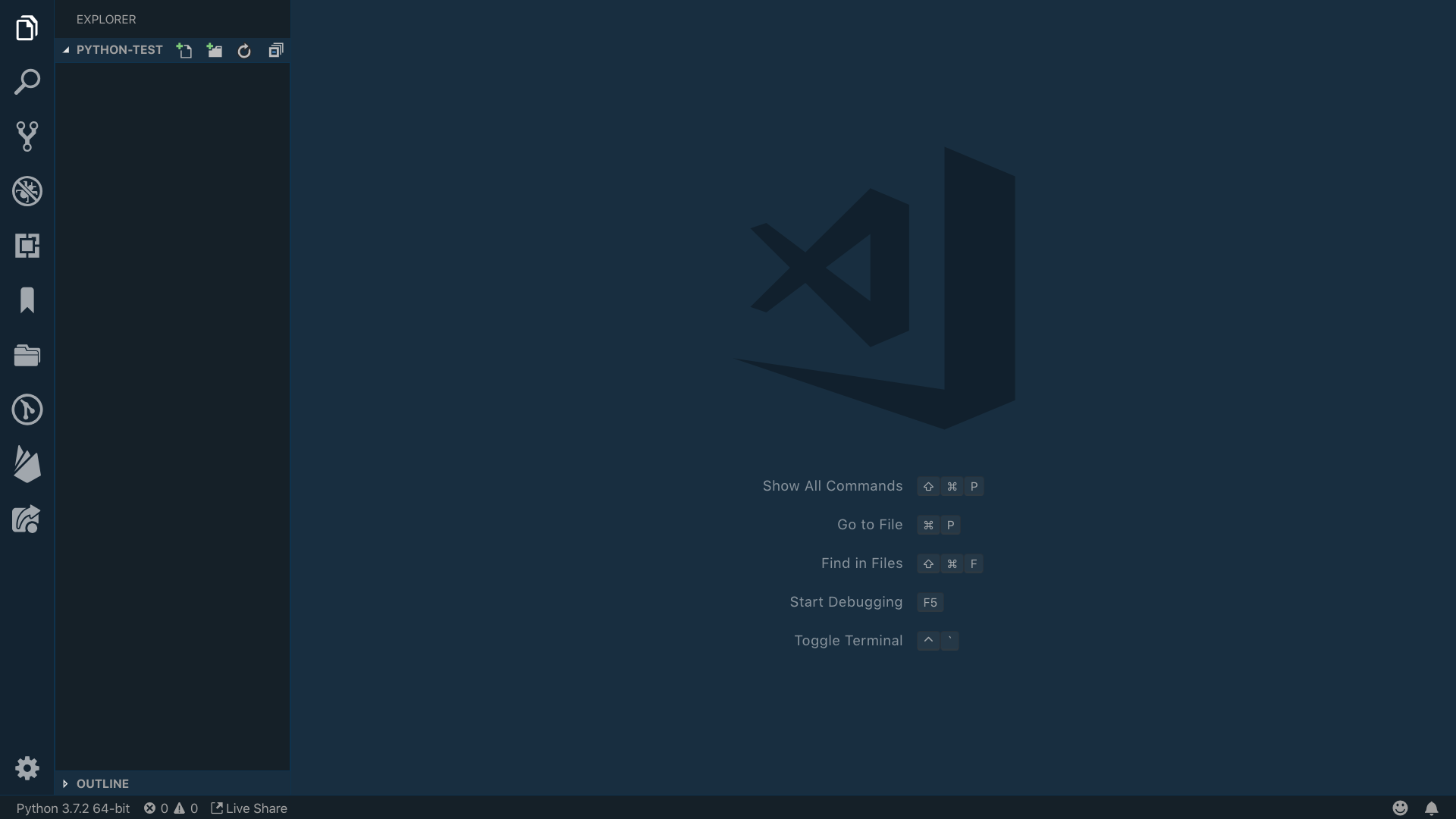
In fact, these rules are so powerful that they actually allow Conway's Game of Life to be Turing Complete, which roughly means that we could perform any computation within the game that a standard computer could (though they would be painfully slow). These extremely simple rules give rise to some incredibly complex and beautiful patterns, as illustrated here:
#Visual studio python tutorial pdf plus#
A given cell's neighbors are those cells directly above, below, left, or right of the cell, plus with the cells diagonally adjacent to it (the cells touching its diagonals). Our "Game of Life" board will be an n-by-n grid of square cells, each of which can be either "alive" or "dead".
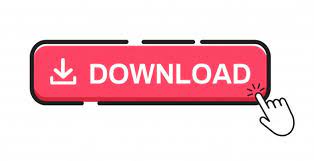